Javascript에서는 pass by reference가 없고, pass by value만 있다. value는 복사되고 이 복사된 value가 argument로서 넘겨지는 것이다. 복사된 것을 바꿀 수 있지만 원래 value는 그대로 유지된다. 예를 들어, function changeValue(a) { a = 5; console.log(a); // 5 } const a = 1; changeValue(a); console.log(a); // 1 function changeValue에서 a는 5로 변경된 것 처럼 보이겠지만, 실제로 a는 변경되지 않았다. 변경된 것은 a의 복사본이다. object도 또한 pass by value인데, 여기에는 차이가 있다. object는 메모리에 참조(reference)할..
Rest syntax는 마지막 변수 이후의 array 의 나머지 모든 elements를 모은다. skip된 elements를 포함하지 않는다. const mainMenu = ["Pizza", "Pasta", "Risotto"]; const starterMenu = ["Focaccia", "Bruschetta", "Garlic Bread", "Caprese Salad"]; const [pizza, , risotto, ...others] = [...mainMenu, ...starterMenu]; pizza // "Pizza" risotto // "Risotto" others // ["Focaccia", "Bruschetta", "Garlic Bread", "Caprese Salad"] 따라서 Rest ele..
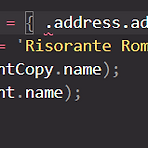
평소에는 불편함 없이 사용하다가 ... 를 입력해야 할 때 자꾸 첫 번째 자동완성 추천 단어가 자동으로 입력되어 불편함을 겪었다. Accept Suggestion On Commit Character 에 체크 해제 해 주면 해결된다. 출처 https://stackoverflow.com/questions/48609354/visual-studio-code-autocomplete-when-i-press-key-any-way-to-disable
앞서 다루었던 restaurant 이름의 object에 orderDelivery라는 이름의 속성을 추가하여 살펴보자!😊 orderDelivery: function(obj) { console.log(obj); }, }; 하나의 argument를 전달받을 수 있는 function restaurant.orderDelivery({ time: '22:30', address: 'Address', mainIndex: 2, starterIndex: 2, }); // 하나의 object를 argument로 전달한 것이다. 이를 참고로 위의 orderDelivery를 아래와 같이 수정해보자 orderDelivery: function({ starterIndex, mainIndex, time, address }){ conso..
지난 포스팅의 restaurant object에 openingHours를 추가하여 진행해보자! const restaurant = { name: 'Restaurant Name', location: 'Seoul', categories: ['Italian', 'Pizzeria', 'Vegeterian', 'Organic'], starterMenu: ['Foccacia', 'Bruschetta', 'Garlic Bread', 'Caprese Salad'], mainMenu: ['Pizza', 'Pasta', 'Risotto'], openingHours: { thu: { open: 12, close: 22, }, fri: { open: 11, close: 23, }, sat: { open: 0, close: 24..
기본적으로 배열에서 destructuring을 수행한다고 하면 가장 쉽게 이런 방법을 생각 할 수 있다. const arr = [1, 2, 3]; const a = arr[0]; const b = arr[1]; const c = arr[2]; 이렇게 하나하나씩 첫 번째 항목, 두 번째 항목, 세 번째 항목을 각 한 줄 씩 대입해 줄 수 있다. 위의 코드는 간략하게 이렇게 줄일 수 있다. const arr = [1, 2, 3]; const [a, b, c] = arr; 위 코드와 같은 결과를 확인할 수 있다. 오브젝트로 연습하기 다음과 같은 오브젝트를 두고 연습해보자!🏋️♂️🏋️♀️ const restaurant = { name: 'Restaurant Name', location: 'Seoul', ca..
scope 어디에 변수가 있는가? 특정 변수에 어디서 접근할 수 있는가? 혹은 접근할 수 없는가? types of scope global scope scopes defined by functions scopes defined by blocks : var const, let Javascript 👉 lexical scoping 코드 어디에 functions와 blocks가 적혀 있는가 scope chain 모든 스코프는 그 스코프 바깥에 있는 변수에 접근할 수있다. 부모에만 접근할 수 있다. 위로만 접근할 수 있다. 특정 스코프의 스코프 체인은 모든 부모스코프의 변수들을 모두 더한것이다. 스코프 체인은 functions이 호출된 순서와 전혀 관계가 없다. variable lookup 현재 스코프에 변수가 없..
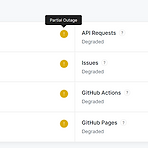
GitHub에 push 하려는데 오류가 계속 발생하는 상황 사용자명@PC명 MINGW64 작업공간 (master) $ git push origin master . .(생략) . remote: fatal error in commit_refs To https://github.com/사용자명/repository명.git ! [remote rejected] master -> master (failure) error: failed to push some refs to 'https://github.com/사용자명/repository명.git' 오늘 처음 겪어본 상황이었다. 처음에는 뭔가 실수한 게 있나 찾아봤는데, 검색을 통해서 찾아봐도 pull후 push하라는 글이 많았는데 repository에서 pull해 올 ..
High-level Garbage-collected Interpreted or just-in-time compiled Multi-paradigm Procedural programming Object-oriented programming (OOP) Functional programming(FP) Prototype-based object-oriented First-class functions Dynamic Single-threaded Non-blocking event loop